Inheritance in GoLang
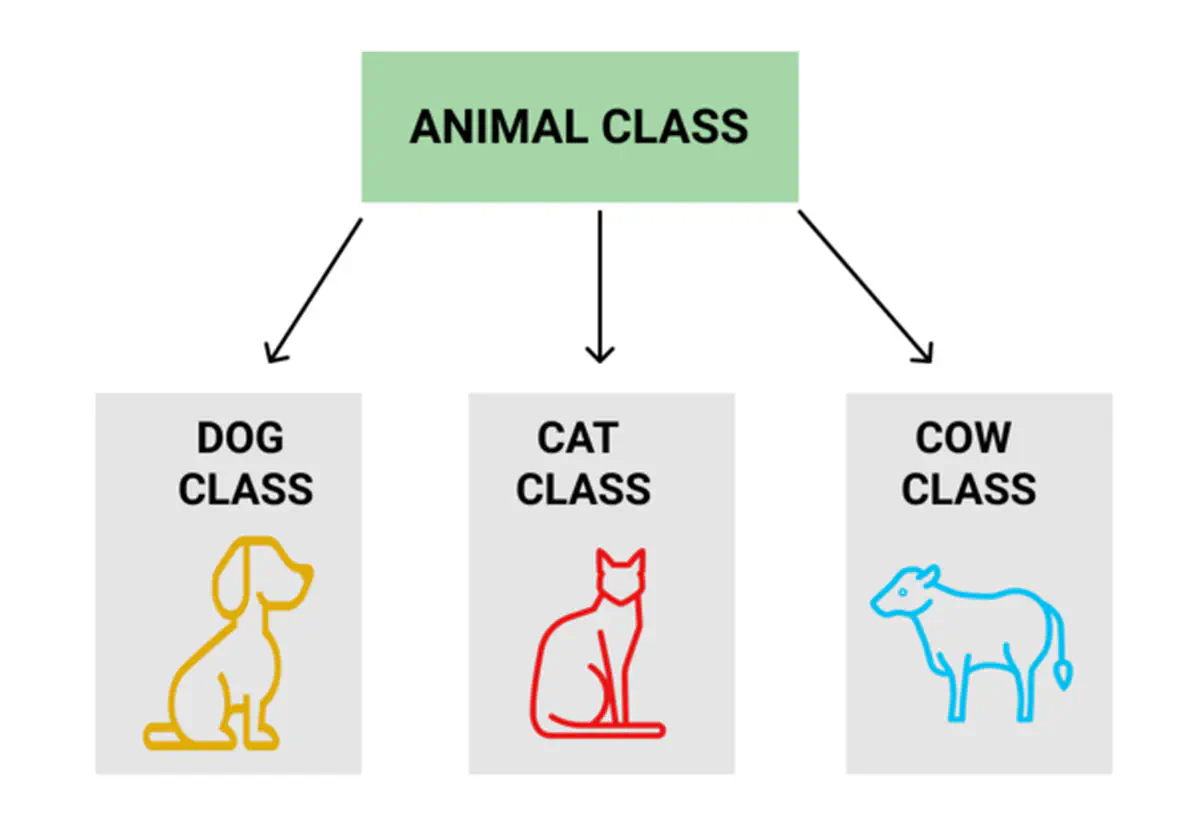
Contents
Inheritance is an important concept in OOP (Object-Oriented Programming). Since Golang does not support classes, there is no inheritance concept in GoLang. However, you can implement inheritance through struct embedding.
In composition, base structs can be embedded into a child struct, and the methods of the base struct can be directly called on the child struct, as shown in the following example.
|
|
You can embed as many base structs as you want